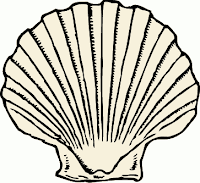
Simple ways to loop in bash shell scripts, or one-liners.
Note that the variable in bash is declared without a "$", and called with it, but in perl the variable is declared with and called with a "$" at the beginning of the name.
The Bash "for" Loop
Loop a number of times
for i in {1..10}; do echo -n "$i ";done;echo
Output:
1 2 3 4 5 6 7 8 9 10
For short lists, the items can be entered manually.
for num in 1 2 3;do echo -n $num;done;echo
Output:
1 2 3
Loop Through Items on a List
See also: How to Run a Bash Command on All Items in a List
for host in `cat all.txt `;do ping -c 1 $host; done
and: Ping Multiple Hosts Using Bash Nmap and Fping
Loop using Perl
This syntax should be familiar to C programmers. In Perl. the scalar variable does not have to be declared as it would in C (int $counter). Note that the variable name starts with "$" to indicate a scalar.
#!/usr/bin/perl
for($counter = 1; $counter <= 10; $counter++){
print "for loop #$counter\n";
}
Wait a minute. Isn't this article about bash and one-liners?
O.K., you can run perl for loops from a one-liner at a bash prompt, or within a shell script.
perl -e for($counter = 1; $counter <= 10; $counter++){
print "for loop #$counter\n";
}
For more detailed scripting ideas, check out the Linux Documentation Project's "Advanced Bash Scripting Guide" -- http://www.tldp.org/LDP/abs/html